You can use JavaScript within Photoshop to automate almost any process. In this example, we’ll use it to open a specific file in a batch workflow (action).
For this quick example, we have 2 folders of image files; 1 folder containing background images and another folder containing text layers. Each set of files follows the same naming convention as follows:
c:\work\backgrounds\image1.tif
c:\work\backgrounds\image2.tif
c:\work\backgrounds\image3.tif
c:\work\text\image1.psd
c:\work\text\image2.psd
c:\work\text\image3.psd
We need to combine the images so that we end up with the text images overlaid on the background.
backgrounds\image1.tif text\image1.psd Combined image
We can run a Photoshop action on the ‘backgrounds’ folder to achieve this. When the action opens a file from the ‘backgrounds’ folder, it can run a JavaScript to open the corresponding image (of the same name) from the ‘text’ folder. This can be really useful if you’ve got hundreds of images to merge.
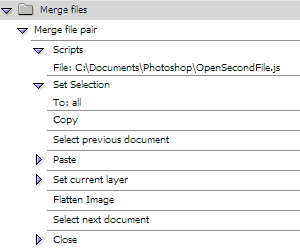
Here’s the JavaScript code that will be used within the action. Edit the variable named secondPath to match your folder names. You can comment out the replace function if you don’t need it in the secondFilename variable.
// Opens a second file with an identical name from a different path
// Turn off display of dialogs
app.displayDialogs = DialogModes.NO;
// Set a variable for the current open document
var firstDoc = app.activeDocument;
// Get the path for the current open document
var firstPath = app.activeDocument.path.toString();
// Create the path for the second document using search/replace
var secondPath = firstPath.replace('backgrounds', 'text');
// Create the second document filename
var secondFilename = app.activeDocument.name.toString().replace('.tif', '.psd');
// Create a variable containing the full path and filename of the second document
var secondFile = File(secondPath + '/' + secondFilename );
try{
// Open the second document
var secondDoc = app.open(secondFile);
// Log to a file
var logFile = new File(firstPath + "/Photoshop-Success.log");
logFile.open("a","TEXT");
logFile.write(activeDocument.name + "\n");
logFile.close();
}catch(e){
// alert ("Second file not found");
// Log to a file
var logFile = new File(firstPath + "/Photoshop-Error.log");
logFile.open("a","TEXT");
logFile.write(activeDocument.name + " -> a matching second file was not found\n");
logFile.close();
// Close first document
activeDocument.close(SaveOptions.DONOTSAVECHANGES);
}
// Set variables to null
firstDoc = null;
firstPath = null;
secondDoc = null;
secondPath = null;
secondFilename = null;
secondFile = null;
layerRef2 = null;
layerRef1 = null;
// Turn on display of dialogs
app.displayDialogs = DialogModes.ALL;