For a time, I had a server with an issue where the IIS SMTP virtual server stopped due to an error. My usual automatic checks for stopped Windows services didn’t pick this up because the Windows service continued to run, even though the SMTP virtual server had stopped.
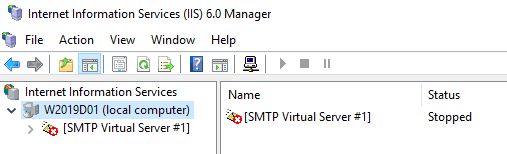
This caused some internal emails to be delayed. It was easy enough to start the virtual server again, but better to put a script in place to check the status and start the SMTP virtual server if necessary.
This PowerShell script did the trick and by running it every 5 minutes as a scheduled task it ensured that there would be no significant email delivery delays until the full solution was found.
#--------------------------------------------------------------------------------
# Script: CheckAndRestartSMTP.ps1
# Author: Dan Tonge, www.techexplorer.co.uk
# Version: 1.0 2015-07-27
# Version: 1.1 2022-02-22: corrected SMTP start command
#
# Information:
# This script checks the status of the Microsoft SMTP virtual server and tries to start it if it's not running
#
# Syntax: .\CheckAndRestartSMTP.ps1
#--------------------------------------------------------------------------------
## include the SendEmail powershell script
."c:\scripts\powershell\Send-Email.ps1"
## build a list of email recipients
$EmailRecipients = @("user@yourdomain.co.uk","admin@yourdomain.co.uk")
$EmailBody = ""
Write-Host("")
Write-Host("Checking the SMTP server status")
Write-Host("")
## define a function to lookup status codes
Function resStatusDescription ($val)
{
Switch ($val){
0 {"Unknown"}
1 {"Unknown"}
2 {"Running"}
3 {"Unknown"}
4 {"Stopped"}
default {"Unknown ($val)"}
}
}
## check the status
$SMTP=[adsi]"IIS://localhost/SMTPSVC/1"
$StatusDescription = resStatusDescription($SMTP.ServerState)
If ($StatusDescription -ne "Running") {
Write-Host($StatusDescription) -Fore Red
## try to start the virtual server
Write-Host("Trying to start the SMTP server")
([adsi]"IIS://localhost/SMTPSVC/1").Start()
## check the status again
$SMTP=[adsi]"IIS://localhost/SMTPSVC/1"
$StatusDescription = resStatusDescription($SMTP.ServerState)
If ($StatusDescription -ne "Running") {
Write-Host($StatusDescription) -Fore Red
$EmailBody = "The SMTP server was not running when this script checked and could not be started."
$EmailBody = $EmailBody + "`nStatus: " + $StatusDescription
## Send email using external mail server
Send-Email -EmailTo $EmailRecipients -EmailSubject "ALERT: SMTP server could not be started" -EmailBody $EmailBody -EmailSMTPServer "smtp.office365.com"
} else {
Write-Host($StatusDescription) -Fore Green
$EmailBody = "The SMTP server was not running when this script checked but has now been started successfully."
$EmailBody = $EmailBody + "`nStatus: " + $StatusDescription
## Send email using external mail server
Send-Email -EmailTo $EmailRecipients -EmailSubject "WARNING: SMTP server had to be started" -EmailBody $EmailBody -EmailSMTPServer "smtp.office365.com"
}
} else {
Write-Host($StatusDescription) -Fore Green
}
Write-host("")
Note that this script uses the Send-Email.ps1 script from this post: https://www.techexplorer.co.uk/windows/powershell-script-to-send-email-with-optional-attachments/
$SMTP.ServerState = 2
$SMTP.SetInfo()
This doesn’t actually start SMTP, it simply makes it look like it’s running. To actually start the service the correct command is:
([ADSI]’IIS://LOCALHOST/SMTPSVC/1′).Start()
Good catch, thanks! Have updated the post now with the correct code.